Write code, not tests
Write code, not tests
Write code, not tests
Celp generates contextually relevant unit tests for Typescript node.js projects, saving you hours
RUN ↓ TO GENERATE YOUR FIRST TESTS
Quick Start:
1. Stage your changes
2. Install celp: npm install -g @celp/celp-cli
3. (Optional) Enhance tests: celp-cli onboard
4
Quick Start:
1. Stage your changes
2. Install celp: npm install -g @celp/celp-cli
3. (Optional) Enhance tests: celp-cli onboard
4
read me
✨
describe("Company Routes", function () {
let authToken: string | null = null;
let sampleData: Awaited<ReturnType<typeof prepareSampleData>> | null = null;
beforeEach(async () => {
await prepareTestDatabase();
sampleData = await prepareSampleData();
authToken = sampleData?.user && generateTestUserToken(sampleData.user);
});
it("should successfully upload a logo for a company", async function () {
const logo = Buffer.from("test-logo-content");
const awsServiceStub = sinon
.stub(awsService, "saveImage")
.resolves("test/path/to/logo.png");
const res = await request(app)
.post(`/companies/${sampleData?.company.id}/upload/logo`)
.set("Authorization", `Bearer ${authToken}`)
.attach("logo", logo, "logo.png");
expect(res.status).to.equal(200);
expect(res.body)
.to.have.property("logo")
.that.equals("test/path/to/logo.png");
describe("Company Routes", function () {
let authToken: string | null = null;
let sampleData: Awaited<ReturnType<typeof prepareSampleData>> | null = null;
beforeEach(async () => {
await prepareTestDatabase();
sampleData = await prepareSampleData();
authToken = sampleData?.user && generateTestUserToken(sampleData.user);
});
it("should successfully upload a logo for a company", async function () {
const logo = Buffer.from("test-logo-content");
const awsServiceStub = sinon
.stub(awsService, "saveImage")
.resolves("test/path/to/logo.png");
const res = await request(app)
.post(`/companies/${sampleData?.company.id}/upload/logo`)
.set("Authorization", `Bearer ${authToken}`)
.attach("logo", logo, "logo.png");
expect(res.status).to.equal(200);
expect(res.body)
.to.have.property("logo")
.that.equals("test/path/to/logo.png");
describe("Company Routes", function () {
let authToken: string | null = null;
let sampleData: Awaited<ReturnType<typeof prepareSampleData>> | null = null;
beforeEach(async () => {
await prepareTestDatabase();
sampleData = await prepareSampleData();
authToken = sampleData?.user && generateTestUserToken(sampleData.user);
});
it("should successfully upload a logo for a company", async function () {
const logo = Buffer.from("test-logo-content");
const awsServiceStub = sinon
.stub(awsService, "saveImage")
.resolves("test/path/to/logo.png");
const res = await request(app)
.post(`/companies/${sampleData?.company.id}/upload/logo`)
.set("Authorization", `Bearer ${authToken}`)
.attach("logo", logo, "logo.png");
expect(res.status).to.equal(200);
expect(res.body)
.to.have.property("logo")
.that.equals("test/path/to/logo.png");
describe("Company Routes", function () {
let authToken: string | null = null;
let sampleData: Awaited<ReturnType<typeof prepareSampleData>> | null = null;
beforeEach(async () => {
await prepareTestDatabase();
sampleData = await prepareSampleData();
authToken = sampleData?.user && generateTestUserToken(sampleData.user);
});
it("should successfully upload a logo for a company", async function () {
const logo = Buffer.from("test-logo-content");
const awsServiceStub = sinon
.stub(awsService, "saveImage")
.resolves("test/path/to/logo.png");
const res = await request(app)
.post(`/companies/${sampleData?.company.id}/upload/logo`)
.set("Authorization", `Bearer ${authToken}`)
.attach("logo", logo, "logo.png");
expect(res.status).to.equal(200);
expect(res.body)
.to.have.property("logo")
.that.equals("test/path/to/logo.png");
Agentic pattern: Tool use
Intelligently parses all your code
Intelligently builds context of your codebase through parsing with Abstract Syntax Trees and intermediary AI prompting, efficiently gathering only the essential information
describe("Company Routes", function () { let authToken: string | null = null; let sampleData: Awaited<ReturnType<typeof prepareSampleData>
const timestamp = new Date().toISOString(); const email = req.user.email; const message = `Welcome to our service, ${email}!`; await prisma.users.update({ data: { updatedAt: new Date() }, where: { id: req.user.id }, }); return res.json({ message, timestamp, email, });
describe("Company Routes", function () { let authToken: string | null = null; let sampleData: Awaited<ReturnType<typeof prepareSampleData>
const timestamp = new Date().toISOString(); const email = req.user.email; const message = `Welcome to our service, ${email}!`; await prisma.users.update({ data: { updatedAt: new Date() }, where: { id: req.user.id }, }); return res.json({ message, timestamp, email, });
describe("Company Routes", function () { let authToken: string | null = null; let sampleData: Awaited<ReturnType<typeof prepareSampleData>
const timestamp = new Date().toISOString(); const email = req.user.email; const message = `Welcome to our service, ${email}!`; await prisma.users.update({ data: { updatedAt: new Date() }, where: { id: req.user.id }, }); return res.json({ message, timestamp, email, });
describe("Company Routes", function () { let authToken: string | null = null; let sampleData: Awaited<ReturnType<typeof prepareSampleData>
const timestamp = new Date().toISOString(); const email = req.user.email; const message = `Welcome to our service, ${email}!`; await prisma.users.update({ data: { updatedAt: new Date() }, where: { id: req.user.id }, }); return res.json({ message, timestamp, email, });
const timestamp = new Date().toISOString(); const email = req.user.email; const message = `Welcome to our service, ${email}!`; await prisma.users.update({ data: { updatedAt: new Date() }, where: { id: req.user.id }, }); return res.json({ message, timestamp, email, });
describe("Company Routes", function () { let authToken: string | null = null; let sampleData: Awaited<ReturnType<typeof prepareSampleData>
const timestamp = new Date().toISOString(); const email = req.user.email; const message = `Welcome to our service, ${email}!`; await prisma.users.update({ data: { updatedAt: new Date() }, where: { id: req.user.id }, }); return res.json({ message, timestamp, email, });
describe("Company Routes", function () { let authToken: string | null = null; let sampleData: Awaited<ReturnType<typeof prepareSampleData>
const timestamp = new Date().toISOString(); const email = req.user.email; const message = `Welcome to our service, ${email}!`; await prisma.users.update({ data: { updatedAt: new Date() }, where: { id: req.user.id }, }); return res.json({ message, timestamp, email, });
describe("Company Routes", function () { let authToken: string | null = null; let sampleData: Awaited<ReturnType<typeof prepareSampleData>
const timestamp = new Date().toISOString(); const email = req.user.email; const message = `Welcome to our service, ${email}!`; await prisma.users.update({ data: { updatedAt: new Date() }, where: { id: req.user.id }, }); return res.json({ message, timestamp, email, });
describe("Company Routes", function () { let authToken: string | null = null; let sampleData: Awaited<ReturnType<typeof prepareSampleData>
Agentic Pattern: Planning
Focusing on the
essential context
After analyzing the context, celp formulates a detailed and strategic plan to ensure that only the most relevant and useful tests are generated
3 passing (7s)
2 failing
1) Company Routes
should return 400 when logo file is not provided:
yarn test
5 passing (6s)
Company Routes
✔ should successfully upload a logo for a company (76ms)
✔ should return 400 when logo file is not provided
✔ should return 400 when companyId is not provided
✔ should return 404 when company does not exist
3 passing (7s)
2 failing
1) Company Routes
should return 400 when logo file is not provided:
yarn test
5 passing (6s)
Company Routes
✔ should successfully upload a logo for a company (76ms)
✔ should return 400 when logo file is not provided
✔ should return 400 when companyId is not provided
✔ should return 404 when company does not exist
3 passing (7s)
2 failing
1) Company Routes
should return 400 when logo file is not provided:
yarn test
5 passing (6s)
Company Routes
✔ should successfully upload a logo for a company (76ms)
✔ should return 400 when logo file is not provided
✔ should return 400 when companyId is not provided
✔ should return 404 when company does not exist
3 passing (7s)
2 failing
1) Company Routes
should return 400 when logo file is not provided:
yarn test
5 passing (6s)
Company Routes
✔ should successfully upload a logo for a company (76ms)
✔ should return 400 when logo file is not provided
✔ should return 400 when companyId is not provided
✔ should return 404 when company does not exist
Agentic Pattern: Reflection
Automatically runs and resolves tests
Iteratively runs your unit tests locally, automatically diagnosing and resolving any errors or failures
describe("Company Routes", function () {
let authToken: string | null = null;
let sampleData: Awaited<ReturnType<typeof prepareSampleData>> | null = null;
beforeEach(async () => {
await prepareTestDatabase();
sampleData = await prepareSampleData();
authToken = sampleData?.user && generateTestUserToken(sampleData.user);
});
it("should successfully upload a logo for a company", async function () {
const logo = Buffer.from("test-logo-content");
const awsServiceStub = sinon
.stub(awsService, "saveImage")
.resolves("test/path/to/logo.png");
const res = await request(app)
.post(`/companies/${sampleData?.company.id}/upload/logo`)
.set("Authorization", `Bearer ${authToken}`)
.attach("logo", logo, "logo.png");
expect(res.status).to.equal(200);
expect(res.body)
.to.have.property("logo")
.that.equals("test/path/to/logo.png");
Agentic pattern: Tool use
Intelligently parses all your code
Intelligently builds context of your codebase through parsing with Abstract Syntax Trees and intermediary AI prompting, efficiently gathering only the essential information
describe("Company Routes", function () { let authToken: string | null = null; let sampleData: Awaited<ReturnType<typeof prepareSampleData>
const timestamp = new Date().toISOString(); const email = req.user.email; const message = `Welcome to our service, ${email}!`; await prisma.users.update({ data: { updatedAt: new Date() }, where: { id: req.user.id }, }); return res.json({ message, timestamp, email, });
const timestamp = new Date().toISOString(); const email = req.user.email; const message = `Welcome to our service, ${email}!`; await prisma.users.update({ data: { updatedAt: new Date() }, where: { id: req.user.id }, }); return res.json({ message, timestamp, email, });
describe("Company Routes", function () { let authToken: string | null = null; let sampleData: Awaited<ReturnType<typeof prepareSampleData>
Agentic Pattern: Planning
Focusing on the
essential context
After analyzing the context, celp formulates a detailed and strategic plan to ensure that only the most relevant and useful tests are generated
3 passing (7s)
2 failing
1) Company Routes
should return 400 when logo file is not provided:
yarn test
5 passing (6s)
Company Routes
✔ should successfully upload a logo for a company (76ms)
✔ should return 400 when logo file is not provided
✔ should return 400 when companyId is not provided
✔ should return 404 when company does not exist
Agentic Pattern: Reflection
Automatically runs and resolves tests
Iteratively runs your unit tests locally, automatically diagnosing and resolving any errors or failures
Celp vs Copilot
Celp vs Copilot
Celp vs Copilot
let's take a look at how we stack up against the top unit testing tools.
Celp vs Copilot
let's take a look at how we stack up against the top unit testing tools.
Celp
Celp
Celp

understands code structure using abstract syntax trees

Writes type-safe code

Generates unit tests from selection

Generates unit tests from diff

tests pass without much intervention

Reuses existing code and helper methods

automatically runs and fixes tests

Learns as you use it (without training a model)
Celp

understands code structure using abstract syntax trees

Writes type-safe code

Generates unit tests from selection

Generates unit tests from diff

tests pass without much intervention

Reuses existing code and helper methods

automatically runs and fixes tests

Learns as you use it (without training a model)
Github Copilot

understands code structure using abstract syntax trees

writes type-safe code

Generates unit tests from selection
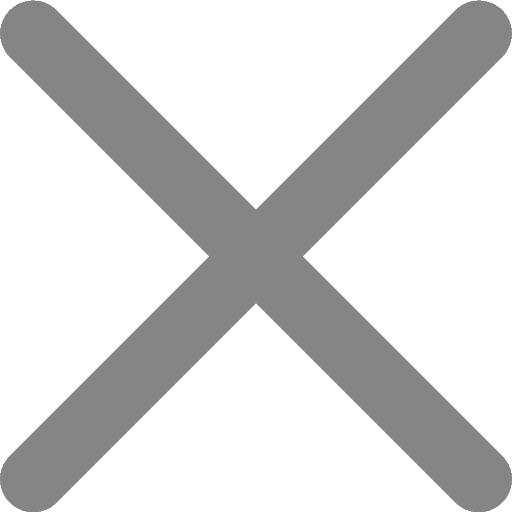
Generates unit tests from diff
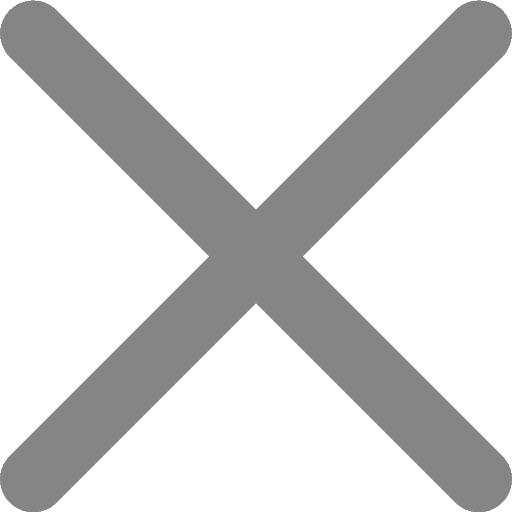
Tests pass without much intervention
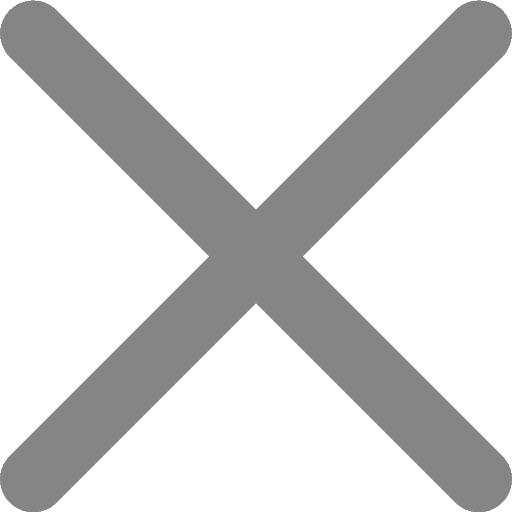
Reuses existing code and helper methods
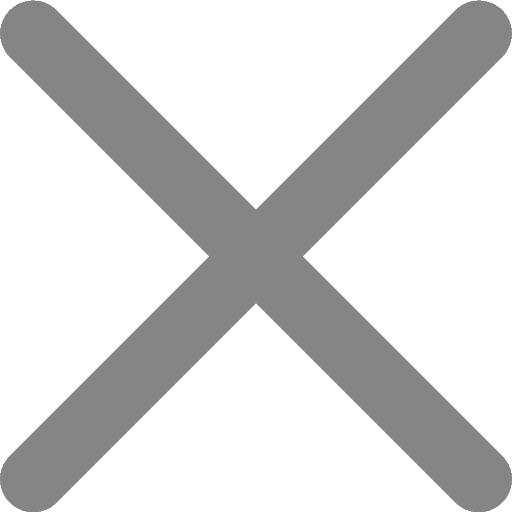
Automatically runs and fixes tests
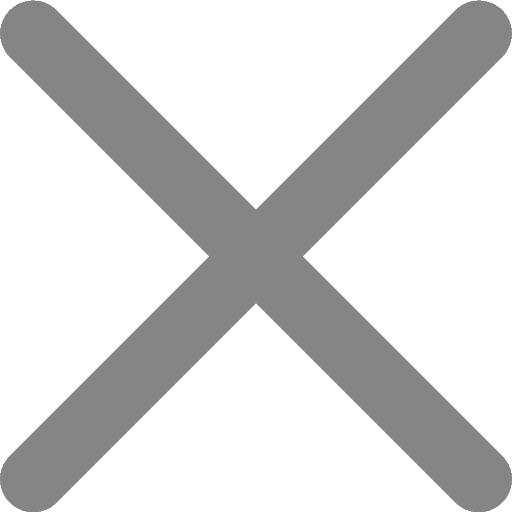
Learns as you use it
Github Copilot

understands code structure using abstract syntax trees

writes type-safe code

Generates unit tests from selection
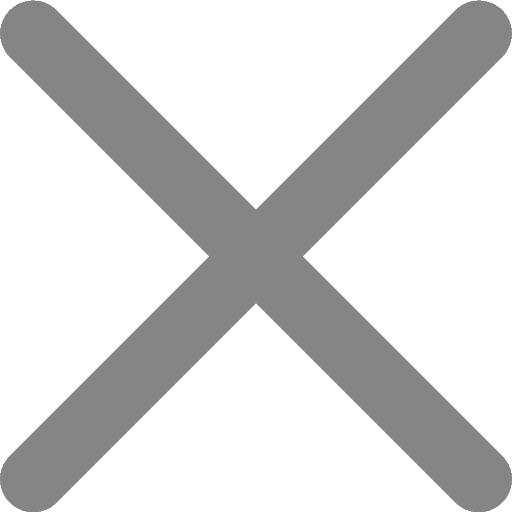
Generates unit tests from diff
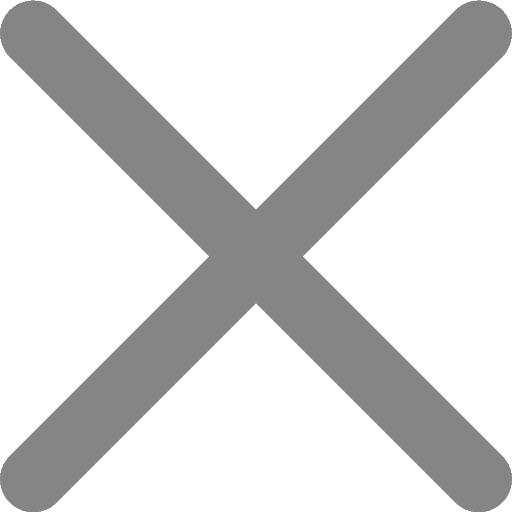
Tests pass without much intervention
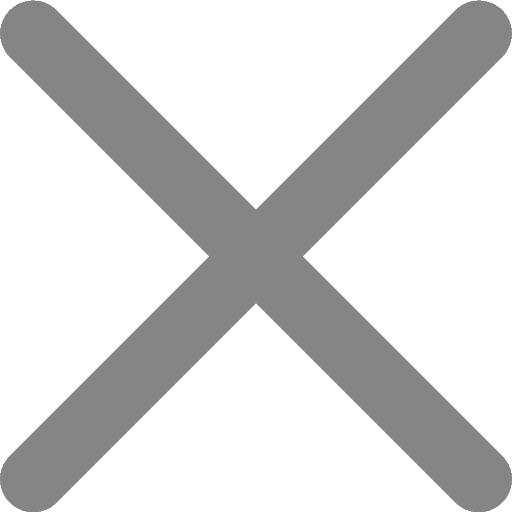
Reuses existing code and helper methods
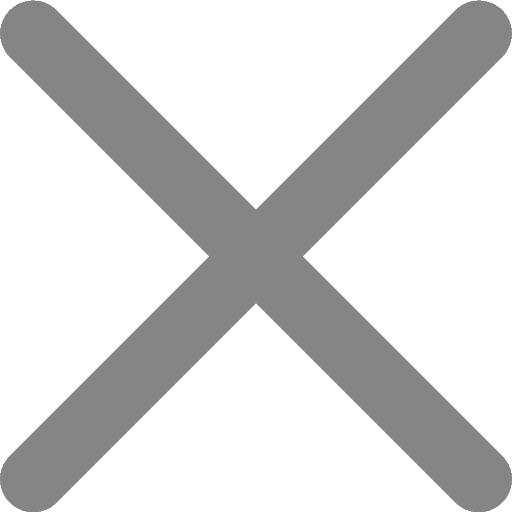
Automatically runs and fixes tests
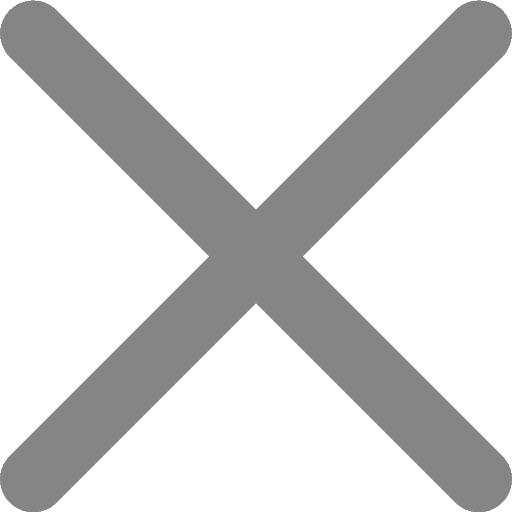
Learns as you use it
Info
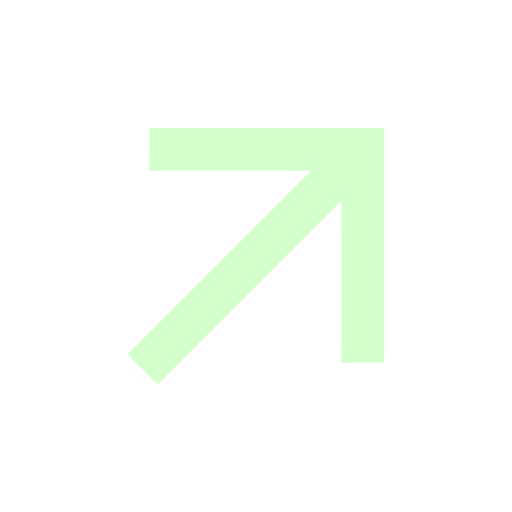
What's unique about Celp?
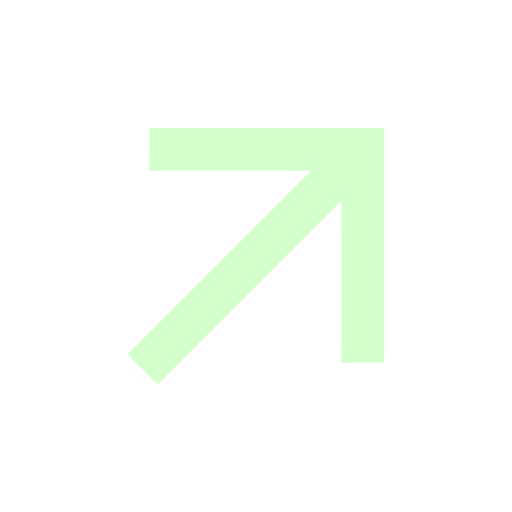
How much does it cost?
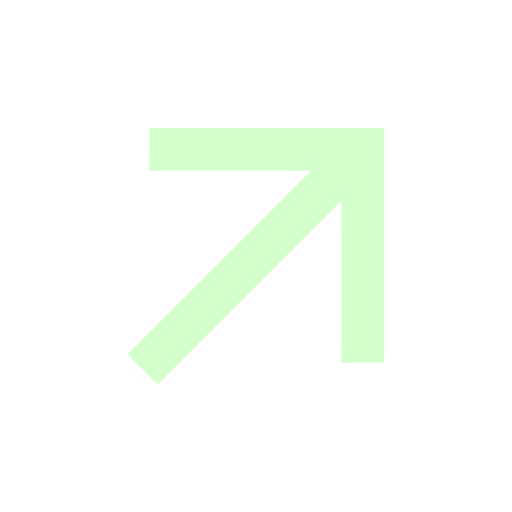
What types of projects does Celp support?
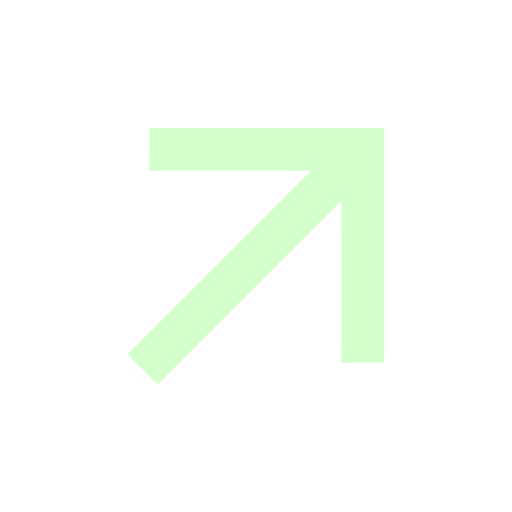
Does Celp keep any code that it uses for context?
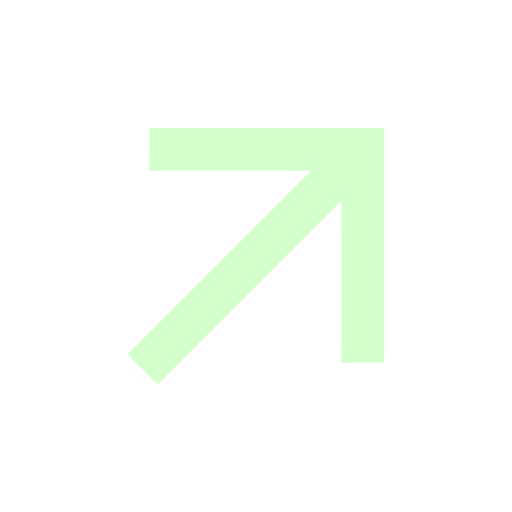
What's unique about Celp?
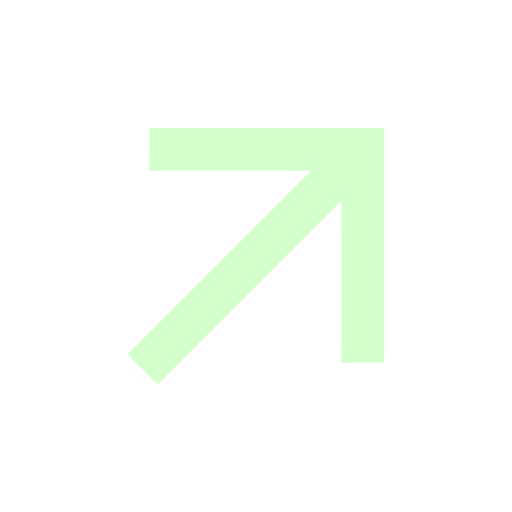
How much does it cost?
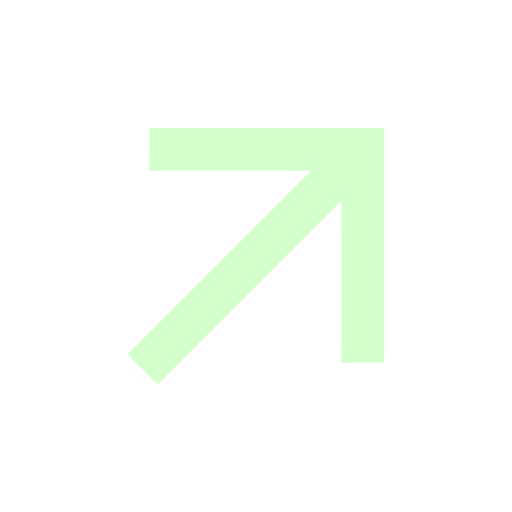
What types of projects does Celp support?
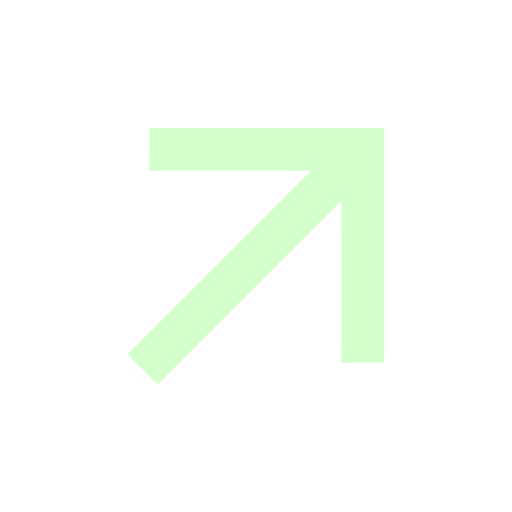
Does Celp keep any code that it uses for context?
Celp vs Copilot
Celp

understands code structure using abstract syntax trees

Writes type-safe code

Generates unit tests from selection

Generates unit tests from diff

tests pass without much intervention

Reuses existing code and helper methods

automatically runs and fixes tests

Learns as you use it (without training a model)
Github Copilot

understands code structure using abstract syntax trees

writes type-safe code

Generates unit tests from selection
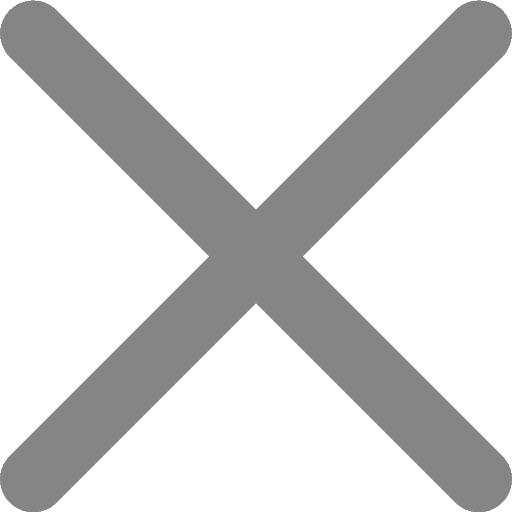
Generates unit tests from diff
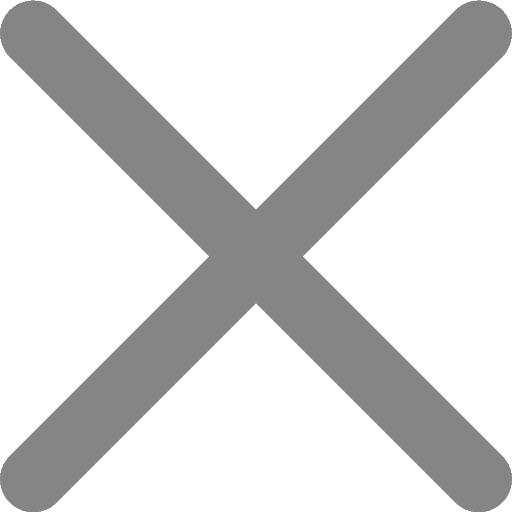
Tests pass without much intervention
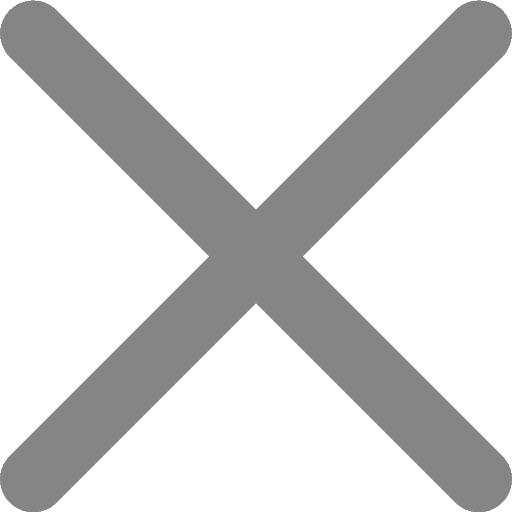
Reuses existing code and helper methods
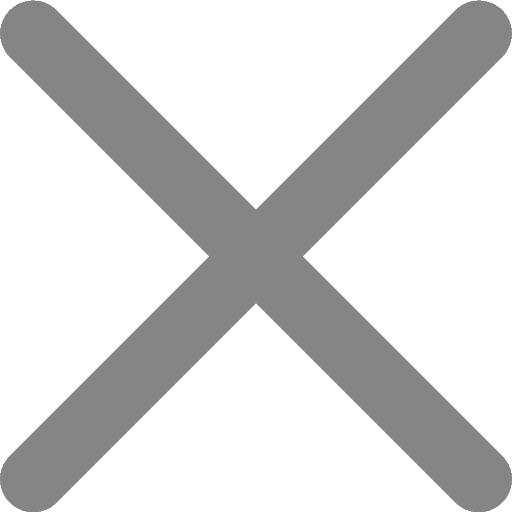
Automatically runs and fixes tests
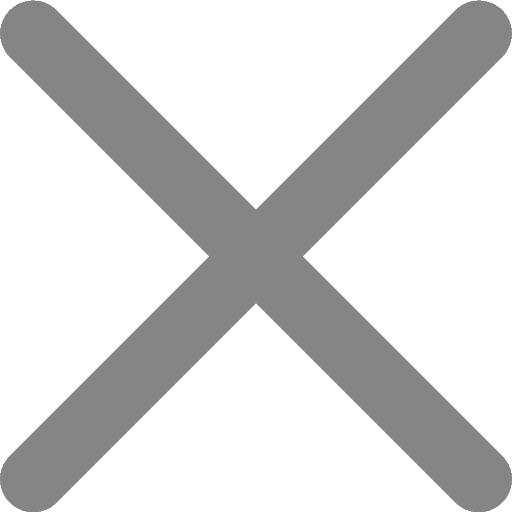
Learns as you use it